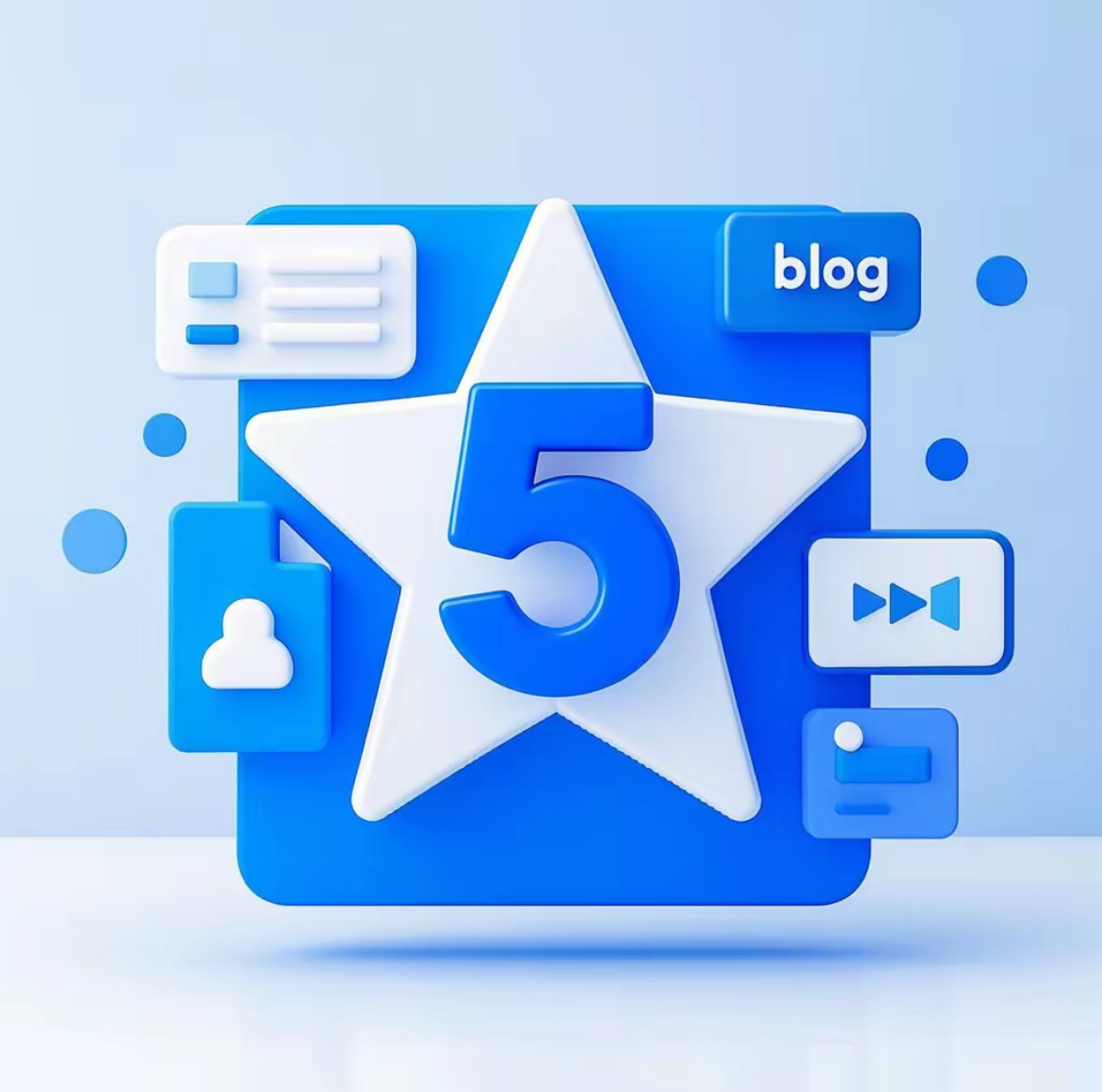
在网络通信中,json是比较普及的数据交换格式了,delphi中有很多这方面的支持,比如QDAC的QJSON和常见的superobject等,rtc自己也有json解析的支持,虽然qjson很好,但我还是继续用rtc的json,这个也可以说是习惯问题吧。
脚本模型中我提供了两个函数,分别是将json转换成记录集和记录集转换成json,比如JsonToRecord转换以后,就可以直接通过$x.count的形式去访问json中的对象了,是不是比较方便?
JsonToRecord('{"name": "姓名","sex": "性别"}');
|<?$x:=JsonToRecord('{"name": "姓名","sex": "性别"}');'all:'$x;?>
|<?'count:'$x.count;?>
|<?'name:'$x('name');?>
|<?'sex:'$x('sex');?>
RecordToJson(JsonToRecord('{"name": "姓名","sex": "性别"}'));
|<?UnicodeToAnsi(RecordToJson(JsonToRecord('{"name": "姓名","sex": "性别"}')));?>
ps:这里引用了UnicodeToAnsi来显示中文